Discovering MicroPython on the Raspberry Pi Pico
(Updated at 12/26/2022)
The easiest thing to do on a new board is to flash the built-in LED. This is the equivalent of the famous Hello World displayed on the terminal. This script will make the built-in LED blink every second. The idea here is to show you an overview of programming in MicroPython.
from machine import Pin
import time
pin_led = Pin(25, mode=Pin.OUT)
while True:
pin_led.on()
time.sleep(1)
pin_led.off()
time.sleep(1)
Note
It’s best to know the basics of Python syntax before jumping into MicroPython.
Running the MicroPython script on your Pico.
First, ensure you have configured your IDE software for your Pico board. If necessary, you can refer to the following tutorial, the procedure to follow for configuring the Raspberry Pi Pico in Thonny IDE .
On Thonny IDE, it is better to save the code on the computer and not directly on the board. Thonny IDE will send the code directly to the MicroPython interpreter (via the REPL) when executing the script. To save the script on the board afterward, go to File → Save as.
Note that the Python script will not automatically launch when the Pico board is switched on . To remedy this, it is necessary to register the script on the board with the following name main.py . This is not very annoying during the development phase, but it is important to know when using a MicroPython script on a board.
A detailed explanation of a MicroPython script
The structure of a MicroPython script is as follows:
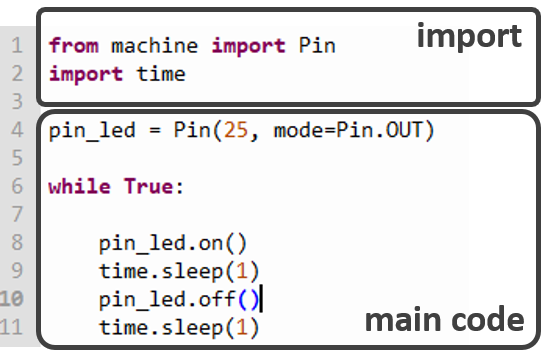
Each MicroPython script is preceded by a phase of importing the modules necessary for its proper functioning. This is equivalent to the #include
Arduino IDE code for external libraries #include <SPI.h>
, #include <SD.h>
..
The difference with MicroPython is that even basic objects must be imported . As a reminder, Python is an object-oriented language: any element in Python is considered an object. For example, the input/output pins of the boards will be seen and manipulated as objects. MicroPython objects are easily used even without being an expert in object-oriented programming.
In the example script, we import from the machine module the sub-module Pin
. The machine
module contains most of the objects specific to MicroPython . The module Pin
is, in fact, a class that defines the operation of an input/output pin (GPIO). This is why we create a pin_led
, corresponding to the pin that controls the built-in LED. This pin is defined as an electrical output. The Pin
object we created has functions to change its state: .on()
turns on the LED, and .off()
turns off the LED.
Note
The number of the pin connected to the integrated LED is 25 for the Raspberry Pi Pico and the uPesy RP2040 DevKit.
We add a one-second delay with the sleep()
function of the time
module to see the blinking visually.
The code that makes the LED blink is contained in an infinite loop.
You will notice if you used to use Arduino code that there are no more setup()
function and loop()
function: the script is executed once in a sequential way. So don’t forget the infinite loop to have a behavior similar to the Arduino sketch.
Improve the current blink script in MicroPython
Thanks to the multiple functions of MicroPython, we can condense the program blink . Indeed, the function .toggle()
allows to invert the state of an output: If the pin is in a high state (3.3V), then the pin is low (0V) after the execution of the function .toggle()
and vice versa.
Thus, the new code (with accelerated flashing) is summarized as:
from machine import Pin
import time
pin_led = Pin(25, mode=Pin.OUT)
while True:
pin_led.toggle()
time.sleep_ms(250)
You will notice that time.sleep()
has been replaced by time.sleep_ms()
for more clarity. In fact, there are 3 functions that allow you to pause the program for a certain time, each with a different level of precision:
time.sleep(duration_in_seconds)
time.sleep_ms(duration_in_milliseconds)
time.sleep_us(duration_in_microseconds)
Note
1 second = \(10^3\) milliseconds = \(10^6\) microseconds
Thus the following three lines will pause the program for 5 milliseconds:
time.sleep(0.005)
time.sleep_ms(5)
time.sleep_us(5000)
Note
As for the Arduino code, these functions will stop the program: it will not be able to execute other tasks in the background, such as reading data from a sensor or measuring a voltage.
You could see an overview of the programming of Raspberry Pi Pico boards with MicroPython. I hope this has made you want to discover more about this new language.