Using the ESP32 capacitive sensors in MicroPython
(Updated at 01/20/2023)
The ESP32 comes with capacitive sensors that can be used instead of conventional push buttons. These are sometimes known as “TOUCH” on ESP32 pinouts found online. There are 10 on the uPesy ESP32 boards that can be utilized with MicroPython.
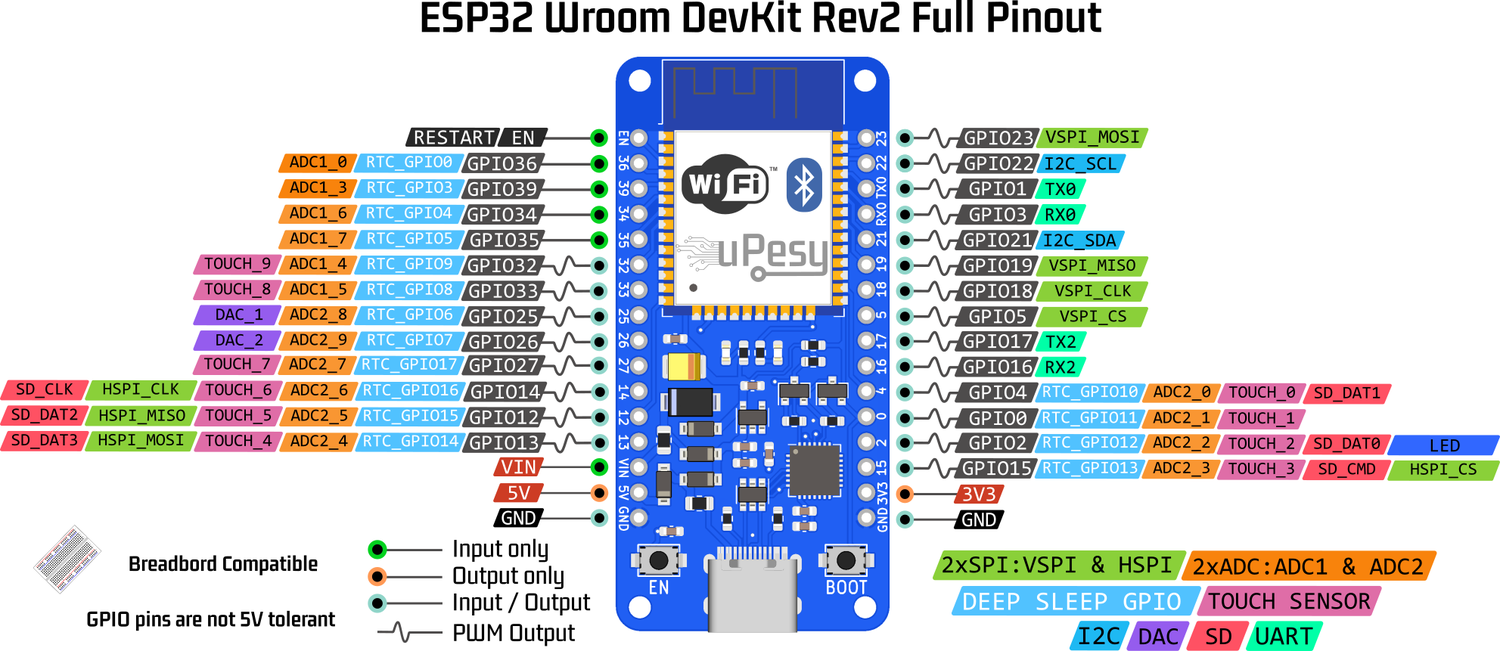
How does a capacitive sensor work?
Capacitive sensors are frequently used to detect the pressure of our fingers on everyday devices, such as touch screens. They are famous for this type of application.
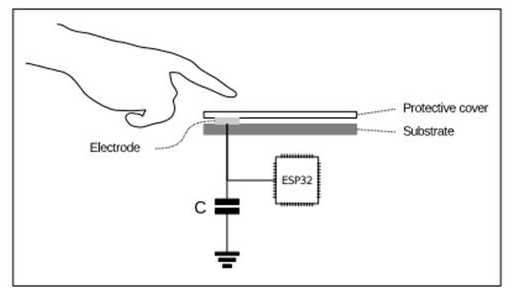
Capacitive touch sensors detect changes in capacitance when touched. A capacitor typically has two metal plates facing each other, separated by a non-conductive substrate. On a capacitive sensor, one plate is fixed, while the other is your finger. The ESP32’s Analog-to-Digital Converter (ADC) converts this change into an analog value.
Note
Capacitive sensors are less reliable than mechanical pushbuttons, which are more prone to environmental noise interference. It is essential to consider this when using them in your projects.
Understanding the values obtained can sometimes be challenging due to interference from outside factors. To gain a better comprehension of this, take a look at this diagram which illustrates the different sources of disturbances.
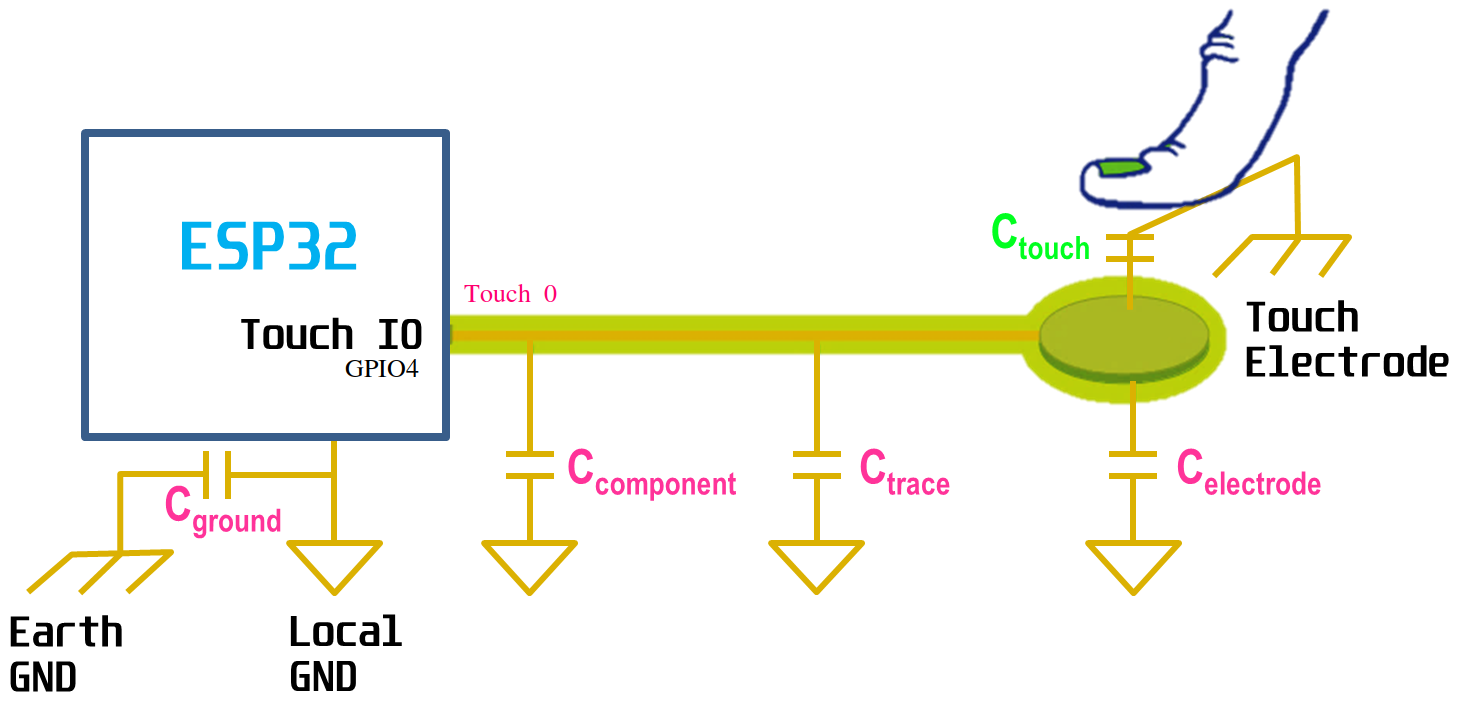
Good results can be achieved by considering all the required parameters and designing an electrical circuit that is more sophisticated than the one provided. A simple copper wire is enough to detect a finger’s pressure.
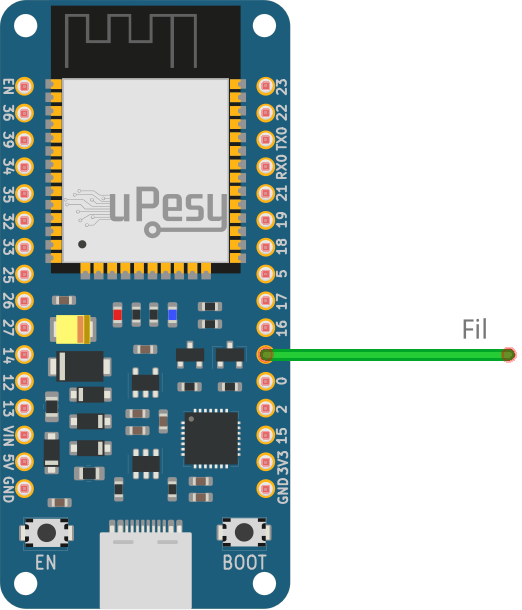
Using the ESP32 capacitive sensors with MicroPython
Micro-Python can measure analog values instead of just binary values of LOW
or HIGH
. The values measured range from 0 to 4095.
Note
We indicate the PIN with a TOUCH
functionality in the Python script. For instance, we use TOUCH0
, connected to the GPIO4
pin.
from machine import TouchPad, Pin
import time
touch_pin = TouchPad(Pin(4, mode=Pin.IN))
while True:
touch_value = touch_pin.read()
print(touch_value)
time.sleep_ms(500)
As for the code, it is identical to that of the ADC, except for the change of ADC(Pin(36, mode=Pin.IN))
to TouchPad(Pin(4, mode=Pin.IN))
.
Analog values must be given a particular reference point (threshold value) to detect pressure. When nothing is pressing on the wire, the value is usually high. When the wire is touched, however, the value drops significantly.
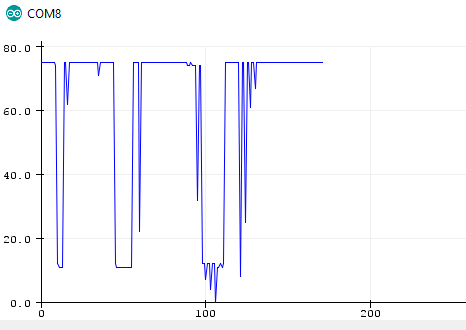
Note
The threshold value depends on the type of material used, such as the wire, length, or breadboard, so you may need to fine-tune the current for your particular application.
Here is an example with a threshold of 150
:
from machine import TouchPad, Pin
import time
capacitiveValue = 500
threshold = 150 # Threshold to be adjusted
touch_pin = TouchPad(Pin(4))
print("\nESP32 Touch Demo")
while True: # Infinite loop
capacitiveValue = touch_pin.read()
if capacitiveValue < threshold:
print("Fil touché")
time.sleep_ms(500)
See for yourself the result :
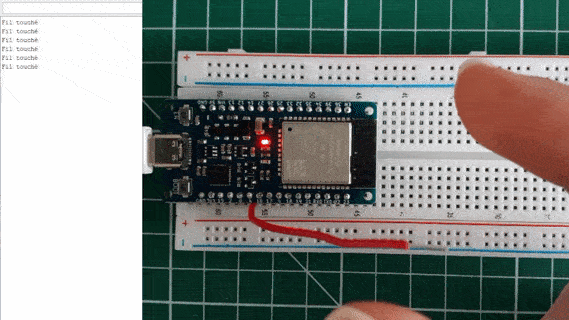
That’s all! There are readily available capacitive sensing modules that contain a circuit that can convert the capacitance levels into binary values.
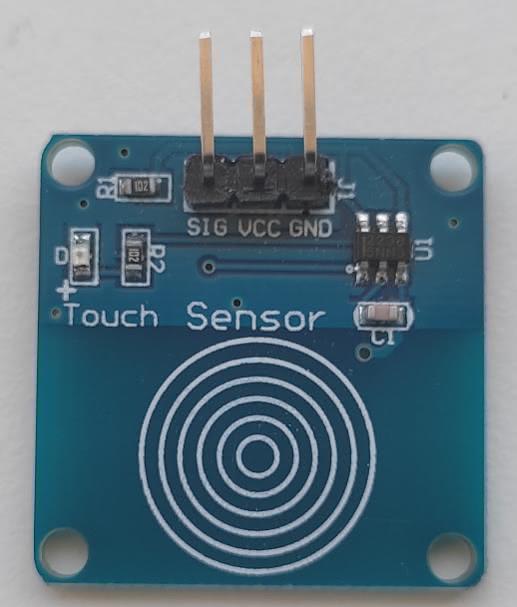
On this side, there is only the conversion circuit, no plate
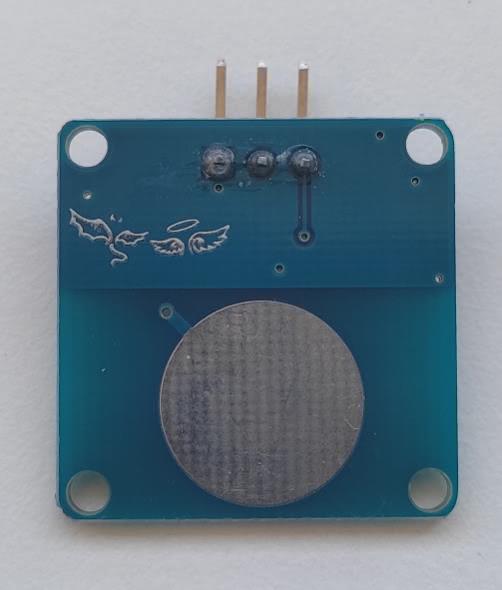
If you want to obtain a signal for your ESP32 to convert, avoid modules that come with a conversion circuit. Instead, opt for modules that provide a raw signal, which the ESP32 can convert using its internal circuit. An example of such a module is a PCB with a designated space for a finger.